Handle Events & Authorization Refresh
Transaction status updates are sent via Event Notification and can also be retrieved via the Trustly Transactions Report. For more information on Event Notifications, refer to Event Notifications.
Capture and Deposit Transactions
The following diagram shows the Transaction State of a Capture or Deposit transaction.
- The Capture and Deposit API creates a transaction with the initial status of
Authorized
. - Once the transaction is submitted to the network for processing, the transaction is moved to the
Processed
state. - After 3 banking days, if the transaction has not been moved to the
Denied
state, it is moved to theCompleted
state. - If there are any issues depositing the funds after the transaction has been moved to
Completed
, it is moved to theReversed
state.
Refund and Reclaim Transactions
The following diagram shows the Transaction State of a Refund or Reclaim transaction. Transaction status updates are sent via Event Notification and can also be retrieved via the Trustly Transactions Report.
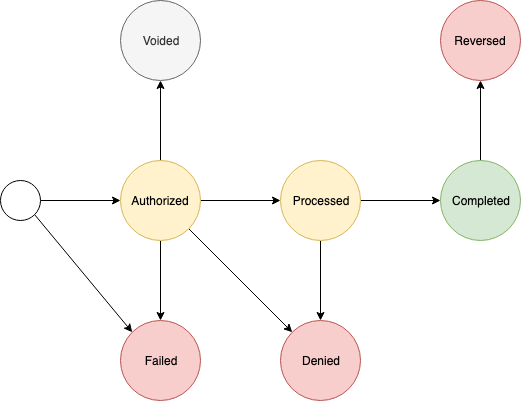
- The Refund and Reclaim API creates a transaction with the initial status of
Authorized
. - Once the transaction is submitted to the network for processing, the transaction is moved to the
Processed
state. - After 3 banking days, if the transaction has not been moved to the
Denied
state, it is moved to theCompleted
state. - If there are any issues depositing the funds after the transaction has been moved to
Completed
, it is moved to theReversed
state.
Handling an Adverse Action decline
If you receive a Capture API or Deposit API response or receive an Event Notification with a Payment Provider Transaction Status of SW055
- Fraud analysis (Negative Data) and the response also contains a thirdPartyDeclineCode
value, it means the user has an item on file with TeleCheck that needs to be resolved, and the user must contact TeleCheck directly. In this instance, you must provide this message to your user, replacing {{ thirdPartyDeclineCode }}
with the thirdPartyDeclineCode
value that was provided in the response or notification.
Note
This use case can be tested using Demo Bank and the
tckerror
password in our Sandbox environment. Select the SW055 account and execute your Capture call as normal to trigger the Adverse ActionSW055
decline via webhook.
Available Funds Guidance Beta
If your application is configured for Available Funds Guidance, a Fail
or Denied
event may contain a suggestedRetryAmount
property if the event was caused by a risk of insufficient funds. In this case, your application can prompt the user to attempt a new payment using the value of the suggestedRetryAmount
as an upper bound.
Visuals Below are Examples Only
The Trustly UI libraries do not handle Available Funds Guidance. The images below are simply concepts for your application design.
An example of a Deny
event is below. For more information see the Event Notifications reference.
{
"merchantId": "00123",
"merchantReference": "unqiueTransactionIdentifier",
"paymentType": "2",
"transactionType": "3",
"eventId": "1030845856",
"eventType": "Deny",
"objectId": "1025228290",
"objectType": "Transaction",
"message": "Not enough balance",
"timeZone": "Etc/UTC",
"createdAt": "1714503865362",
"errorCode": "331",
"paymentProviderTransaction.reasonCode": "12",
"paymentProviderTransaction.reasonCodeMessage": "Not enough balance",
"paymentProviderTransaction.status": "SW021",
"paymentProviderTransaction.statusMessage": "Not enough balance",
"status": "8",
"statusMessage": "Denied",
"suggestedRetryAmount": "500.00"
}
Note that the original transaction which was denied has failed and can be discarded. Use the Capture API with the original authorization transactionId
to create a new payment transaction.
Refresh a Bank Authorization using Online Banking
Handling invalidated Transaction IDs
If you receive a Capture API response or receive an Event Notification with an error code of 330
and a Payment Provider Transaction Status of SW056
, simply follow these steps:
- Remove the Account from the User's profile.
- Message the User with an appropriate message, such as
Sorry, we are unable to process your request at this time. Please choose a different payment method.
. Allow the User to select another method of payment. - Create a new Bank Authorization using Online Banking. Save the new Authorization on the User's Profile.
Note
This use case can be tested using Demo Bank and the
tckerror
password in our Sandbox environment. Select the SW056 account and execute your Capture call as normal to trigger theSW056
decline via webhook.
Handling expired Split Tokens
If you receive a Capture API response or receive an Event Notification with an error code of 326
and a Payment Provider Transaction Status of SW057
, or an error code of 380
and a Payment Provider Transaction Status of SW051
, or an error code of 397
within an HTTP400
response, simply follow these steps:
- Prompt the User to refresh their Bank Authorization using Online Banking.
- Once you receive the refreshed Split Token via Event Notification, update the User's Profile, storing the updated Split Token.
- Attempt to process the Capture API call again.
Note
This use case can be tested using Demo Bank and the
ExpiredSplitToken
password in our Sandbox environment. Once you successfully create your bank authorization, use the Capture API to trigger theSW057
response.
Refresh an expired Bank Authorization using Online Banking
In the event a User's Split Token expires or becomes invalidated, you can use the Refresh flow to request a new one.
- You call the Trustly SDK's
establish
function, passing in parameters to launch the Refresh flow. - From the Trustly Lightbox, the user authenticates with their bank and is immediately returned to your
returnUrl
. - Before returning the User to your
returnUrl
, we will send an Event Notification to your listener with thetransactionId
andsplitToken
. You'll update the User's account on file with the newsplitToken
at this point and attempt to process the Capture transaction against the original deferred authorizationtransactionId
(not thetransactionId
created from thepaymentType=Verification
refresh flow) again.
To integrate the Trustly Refresh flow into your website or app, you will need to do the following:
Integrate the Trustly SDK into your flow.
Trustly offers 3 SDK's: JavaScript, iOS, and Android. The SDK has 2 main methods: selectBankWidget
and establish
. The methods accept 2 parameters, options
and establishData
. The options
parameter is optional and can be used to control pieces of the Lightbox experience. The establishData
parameter is used to pass transaction parameters to Trustly that are used when establishing the Bank Authorization transaction.
The following examples are using the JavaScript SDK
1. To load the SDK on the page, use the following JavaScript tag (replacing {accessId}
with the Access Id provided to you by Trustly):
<script src="https://sandbox.trustly.one/start/scripts/trustly.js?accessId={accessId}"> </script>
2. To provide optional Trustly configuration options, create a TrustlyOptions
object:
var TrustlyOptions = {
closeButton: false,
dragAndDrop: false,
};
For details on the Trustly configuration options, refer to the SDK Specification.
3. To provide the transaction details to the SDK, create an establishData
object:
var establishData = {
accessId: {accessId},
requestSignature: {requestSignature},
merchantId: {merchantId},
description: "transaction description",
currency: "USD",
amount: "0.00",
merchantReference: "merchant reference",
paymentType: "Verification",
authToken: "new",
transactionId: {transactionId},
returnUrl: "https://merchant.com/trustly/return",
cancelUrl: "https://merchant.com/trustly/cancel"
};
Info
Ensure you are passing in the
transactionId
that you have stored on file. ThistransactionId
will not change.
Warning
Do not pass Consumer PII (name, email address, etc) in the
description
field. You can pass Consumer PII in thecustomer
object.
Tip
Ensure you're securing your call by including the
requestSignature
parameter.
4. Finally, call the Trustly SDK's establish
function:
Trustly.establish(establishData, TrustlyOptions);
The following is a full HTML page using the above example.
Info
You'll want to replace
{accessId}
and{merchantId}
with the values provided to you by Trustly. You'll want to replace{transactionId}
with your stored transaction id.
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1" />
<script>
var TrustlyOptions = {
closeButton: false,
dragAndDrop: true,
widgetContainerId: 'widget',
};
</script>
<script src="https://{environmentURL}.one/start/scripts/trustly.js?accessId={accessId}"></script>
</head>
<body style="margin: 0;">
<div id="widget"></div>
</body>
<script>
var establishData = {
accessId: {accessId},
merchantId: {merchantId},
description: 'transaction description',
currency: "USD|CAD",
amount: "0.00",
merchantReference: "merchant reference",
paymentType: "Verification",
authToken: "new",
transactionId: {transactionId},
returnUrl: "https://merchant.com/trustly/return",
cancelUrl: "https://merchant.com/trustly/cancel"
};
Trustly.establish(establishData, TrustlyOptions);
</script>
</html>
Receive the refreshed Split Token
Before the User is returned via the returnUrl
, Trustly will send you an Event Notification that contains the parentObjectId
(transactionId
), splitToken
, and other related fields. Once you receive the Notification, save the payment method to the User's account and return a 200 OK
response to the Notification server.
Info
Trustly will not redirect the User to your
returnUrl
until we either receive a response (success or failure) or until the request times out after 3 seconds.
Warning
If you fail to receive or store the
splitToken
, it can not be resent. You can (and should) attempt a Capture API call without it.
Example Verification notification
merchantId=1002463580&merchantReference=123123&paymentType=5&transactionType=1&parentObjectId=1002596444&parentMerchantReference=123123&eventId=1002642360&eventType=Authorize&objectId=1002642325&objectType=Transaction&message=&timeZone=Etc%2FUTC&createdAt=1557803299069&accessId=ASs345fldAHJPTHXcaK&accountVerified=true&fiCode=200005501&paymentProviderType=PWMB&status=2&statusMessage=Authorized&splitToken=CLvfv6KrLRABGGAgACowusCukkxmQ%2B%2BQseknVvMke5bDORHYSXZbLnPtCEPvL24Uae2XF7%2BcaSgIW%2BVC19J%2B
Please refer to Event Notifications for more information on handling Event Notifications.
Handle the redirect
If the User cancels the request, Trustly will direct the User to your provided cancelUrl
. If the User successfully authorizes the request, Trustly will direct the User to your provided returnUrl
.
Once you get a successful redirect to your returnUrl
, check to ensure you've received the Split Token and added the account on file. If you have not, add the account on file with the provided transactionId
and a blank or null splitToken
.
Example Cancel URL
https://merchant.com/trustly/cancel?transactionId=1002632909&transactionType=1&merchantReference=123123&status=7&payment.paymentType=2&panel=1&payment.paymentProviderTransaction.status=UC01&requestSignature=tp%2B%2B%2BI5nM%2BSeOT8TQKLGvfaEGcs%3D
Example Return URL
https://merchant.com/trustly/return?merchantReference=123123&payment.account.verified=true&payment.paymentProvider.type=1&payment.paymentType=5&requestSignature=2yBF8Hlrte2yXttLEI934MfOiG4%3D&status=2&transactionId=1002642325&transactionType=1
Redirect URL Parameters
Trustly will append the following parameters to your returnUrl
or cancelUrl
:
Parameter | Definition |
---|---|
transactionId | A unique Trustly transaction identifier. (15 characters) |
transactionType | Will always be 1 in this use case. |
merchantReference | A specific merchant reference for this cancelation. For example, this could be your order number or session id. |
status | Integer value representing the Transaction Status. This will either be 2 (Authorized) or 7 (Cancelled). Refer to Transaction Status Values in the SDK Specification for a complete list of values and their definitions. |
payment.paymentType | Will always be 2 (Deferred) in this use case. |
payment.paymentProvider.type | Will always be 1 (Online Banking) in this use case. |
payment.account.verified | ... |
panel | Integer value representing the Trustly screen the user exited the flow on. Refer to Panel Values in the SDK Specification for a complete list of values and their definitions. |
payment.paymentProviderTransaction.status | Integer value representing the Payment Provider Transaction Status of the transaction. Refer to Payment Provider Transaction Status in the SDK Specification for a complete list of values and their definitions. |
requestSignature | This is a signature that you can calculate to ensure the request you receive is coming from Trustly. See Validate the Redirect Signature for more information. |
Updated about 1 month ago