Instant Payouts
Trustly can be used to allow a user to initiate an instant payout from your application. There are 4 pieces required for this use case.
- Create a Bank Authorization using Online Banking. Implement the Trustly Online Banking interface to allow a User to authenticate with their Bank and authorize you to use that bank for payment transactions. This returns a
transactionId
that you will use to make API calls with Trustly to further process the transaction. - Display Bank or User PII from Trustly. With a valid
transactionId
, you can use the Trustly Get Transaction API and Get Financial Institution User API to retrieve information from the User's selected bank to display, verify, or pre-fill fields on your site or application. - Create a Transaction from the Bank Authorization. When the User requests to make a payment, you can use the Trustly Deposit API, passing the previously obtained
transactionId
,amount
, andmerchantReference
. - Creating Deferred Transactions with the Deposit API. Use the Trustly Deposit Transaction to initiate a Payout transaction to a user's bank account.
Integration Options and Branding Requirements
Trustly offers two types of Integration Options for its Online Banking solution: Select Bank Widget and Trustly Lightbox.
The Select Bank Widget is shown in-line on your page and shows the most popular bank accounts. Selecting one of the buttons on the Widget opens the Trustly Lightbox, where the User can sign in and authorize their account for use.
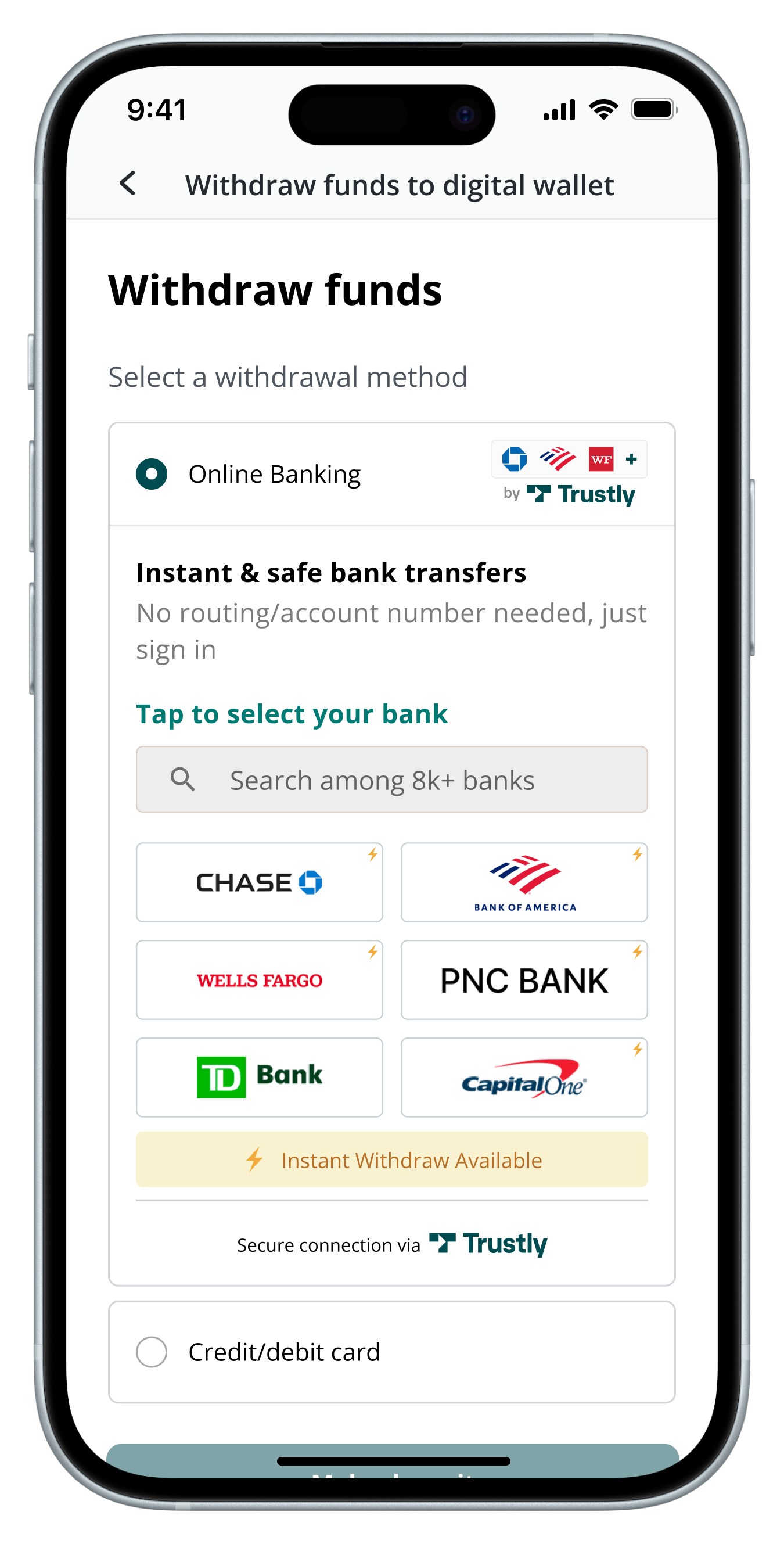
Alternatively, you can trigger the Trustly Lightbox using your own button. The Trustly Lightbox opens over your existing page.
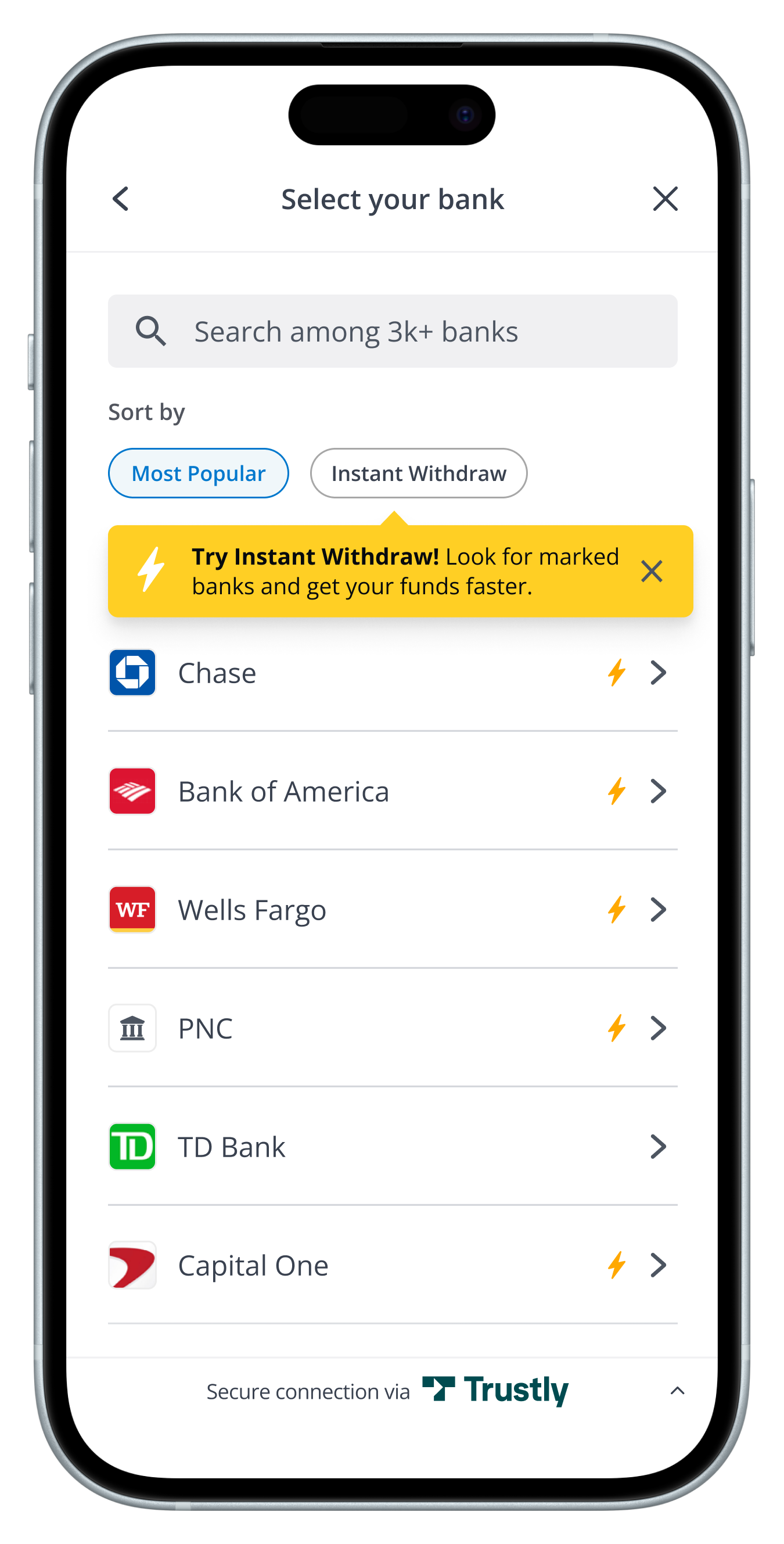
Consider Trustly's Branding Requirements when determining whether the Trustly Lightbox or the Select Bank Widget is right for you. If you have any questions or specific requirements, please work with your Trustly team or contact [email protected].
Create a Bank Authorization using Online Banking
The Trustly Online Banking Payment service enables end-users to pay by signing in to their online banking interface within your website. The Trustly User interaction can be completed in 3 simple steps:
- The User selects Online Banking as a payment method on your website, which either displays the Trustly Widget (which then launches the Trustly Lightbox) or launches the Trustly Lightbox directly.
- From the Trustly Lightbox, the user authenticates with their bank and selects the account they wish to use for the transaction.
- Once authorized, the User is then returned to your
returnUrl
with thetransactionId
, at which point you can continue your flow as usual.
Integrate the Trustly SDK into your flow.
Trustly offers 3 SDK's: JavaScript, iOS, and Android. The SDK has 2 main methods: selectBankWidget
and establish
. The methods accept 2 parameters, options
and establishData
. The options
parameter is optional and can be used to control pieces of the Lightbox experience. The establishData
parameter is used to pass transaction parameters to Trustly that are used when establishing the Bank Authorization transaction.
The following examples are using the JavaScript SDK
1. To load the SDK on the page, use the following JavaScript tag (replacing {accessId}
with the Access Id provided to you by Trustly):
<script src="https://sandbox.trustly.one/start/scripts/trustly.js?accessId={accessId}"> </script>
2. To provide optional Trustly configuration options, create a TrustlyOptions
object:
var TrustlyOptions = {
closeButton: false,
dragAndDrop: false,
widgetContainerId: "widget-container-id" //Page element container for the widget
};
For details on the Trustly configuration options, refer to the SDK Specification.
3. To provide the transaction details to the SDK, create an establishData
object:
var establishData = {
accessId: {accessId},
requestSignature: {requestSignature},
merchantId: {merchantId},
description: "transaction description",
currency: 'USD', // or "CAD"
amount: '0.00',
merchantReference: 'merchant reference',
paymentType: 'Deferred',
customer: {
externalId: '12345',
name: 'Joe User',
},
returnUrl: 'https://merchant.com/trustly.com/return',
cancelUrl: 'https://merchant.com/trustly.com/cancel'
};
Gaming Merchants
Electronic Gaming clients are required to pass the
customer
object for verification.
Warning
Do not pass Consumer PII (name, email address, etc) in the
description
field. Consumer PII can be securely provided handled via thecustomer
object.
Tip
Ensure you're securing your call by including the
requestSignature
parameter.
4. Finally, call the Trustly SDK's establish
or selectBankWidget
function:
Select Bank Widget
Trustly.selectBankWidget(establishData, TrustlyOptions);
Establish
Trustly.establish(establishData, TrustlyOptions);
The following is a full HTML page using the above example.
Info
Replace
{accessId}
and{merchantId}
with the values provided to you by Trustly.
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1" />
<script>
var TrustlyOptions = {
closeButton: false,
dragAndDrop: true,
widgetContainerId: 'widget',
};
</script>
<script src="https://sandbox.trustly.one/start/scripts/trustly.js?accessId={accessId}"></script>
</head>
<body style="margin: 0;">
<div id="widget"></div>
</body>
<script>
var establishData = {
accessId: {accessId},
merchantId: {merchantId},
merchantReference: {merchantReference},
description: 'transaction description',
currency: 'USD',
amount: '0.00',
merchantReference: 'merchant reference',
paymentType: 'Deferred',
returnUrl: 'https://merchant.com/trustly.com/return',
cancelUrl: 'https://merchant.com/trustly.com/cancel'
};
Trustly.selectBankWidget(establishData, TrustlyOptions);
</script>
</html>
Handle the redirect
If the User cancels the request, Trustly will direct the User to your provided cancelUrl
. If the User successfully authorizes the request, Trustly will direct the User to your provided returnUrl
.
Example Cancel URL
https://merchant.com/trustly.com/cancel?transactionId=1002632909&transactionType=1&merchantReference=123123&status=7&payment.paymentType=2&panel=1&payment.paymentProviderTransaction.status=UC01&requestSignature=tp%2B%2B%2BI5nM%2BSeOT8TQKLGvfaEGcs%3D
Example Return URL
https://merchant.com/trustly.com/return?transactionId=1002633191&transactionType=1&merchantReference=123123&status=2&instantPayoutAvailable=true&payment.paymentType=2&payment.paymentProvider.type=1&payment.account.verified=false&panel=1&requestSignature=b7yr%2F3qOupPa1B7VeI32PhGQ7C8%3D
Redirect URL Parameters
Trustly will append the following parameters to your returnUrl
or cancelUrl
:
Parameter | Definition |
---|---|
transactionId | A unique Trustly transaction identifier. (15 characters) |
transactionType | Will always be 1 in this use case. |
merchantReference | A specific merchant reference for this cancelation. For example, this could be your order number or session id. |
status | Integer value representing the Transaction Status. This will either be 2 (Authorized) or 7 (Cancelled). Refer to Transaction Status Values in the SDK Specification for a complete list of values and their definitions. |
payment.paymentType | Will always be 2 (Deferred) in this use case. |
payment.paymentProvider.type | Will always be 1 (Online Banking) in this use case. |
payment.paymentProvider.instantPayoutAvailable | Boolean value that determines whether or not instant payouts are available for this transaction. |
payment.account.verified | ... |
panel | Integer value representing the Trustly screen the user exited the flow on. Refer to Panel Values in the SDK Specification for a complete list of values and their definitions. |
payment.paymentProviderTransaction.status | Integer value representing the Payment Provider Transaction Status of the transaction. Refer to Payment Provider Transaction Status in the SDK Specification for a complete list of values and their definitions. |
requestSignature | This is a signature that you can calculate to ensure the request you receive is coming from Trustly. See Validate the Redirect Signature for more information. |
Display Bank or User PII from Trustly
With a valid Bank Authorization, you can use the Trustly Get Transaction API to retrieve information about the User's bank account that can be displayed in their account on your system. You can also use the Trustly Get User API to retrieve personal information (name, address, email, etc) that can be used to pre-fill fields on your flow or verify the information you have already collected from the User.
Use Get Transaction to display Bank information
Calling the Get Transaction API allows you to get transaction details and the current status of a transaction. You call the Get Transaction API by executing a GET
request to the Get Transaction endpoint (/transactions/{transactionId}
), where {transactionId}
is the Bank Account Authorization transaction id.
Example Get Transaction request
https://sandbox.trustly.one/api/v1/transactions/1002548448
The Get Transaction call returns a JSON response with Bank Account data that you can use in your application. Relevant fields from the response include:
payment.account.name
: Name (friendly identifier) of the User's Bank Account.payment.account.type
: Type of Account selected (Checking or Savings).payment.account.accountNumber
: Last 4 digits of the Bank Account number selected.payment.paymentProvider.paymentProviderId
: Trustly Identifier for the Bank selected. You can use this identifier to display the Bank logo to the User.payment.paymentProvider.name
: Name of the Bank the User selected.payment.paymentProvider.instantPayoutAvailable
: Boolean value that determines whether or not instant payouts are available for this transaction.statusMessage
: Status of the transaction. Should beAuthorized
.
You can then use this information to display the selected payout method to your user.
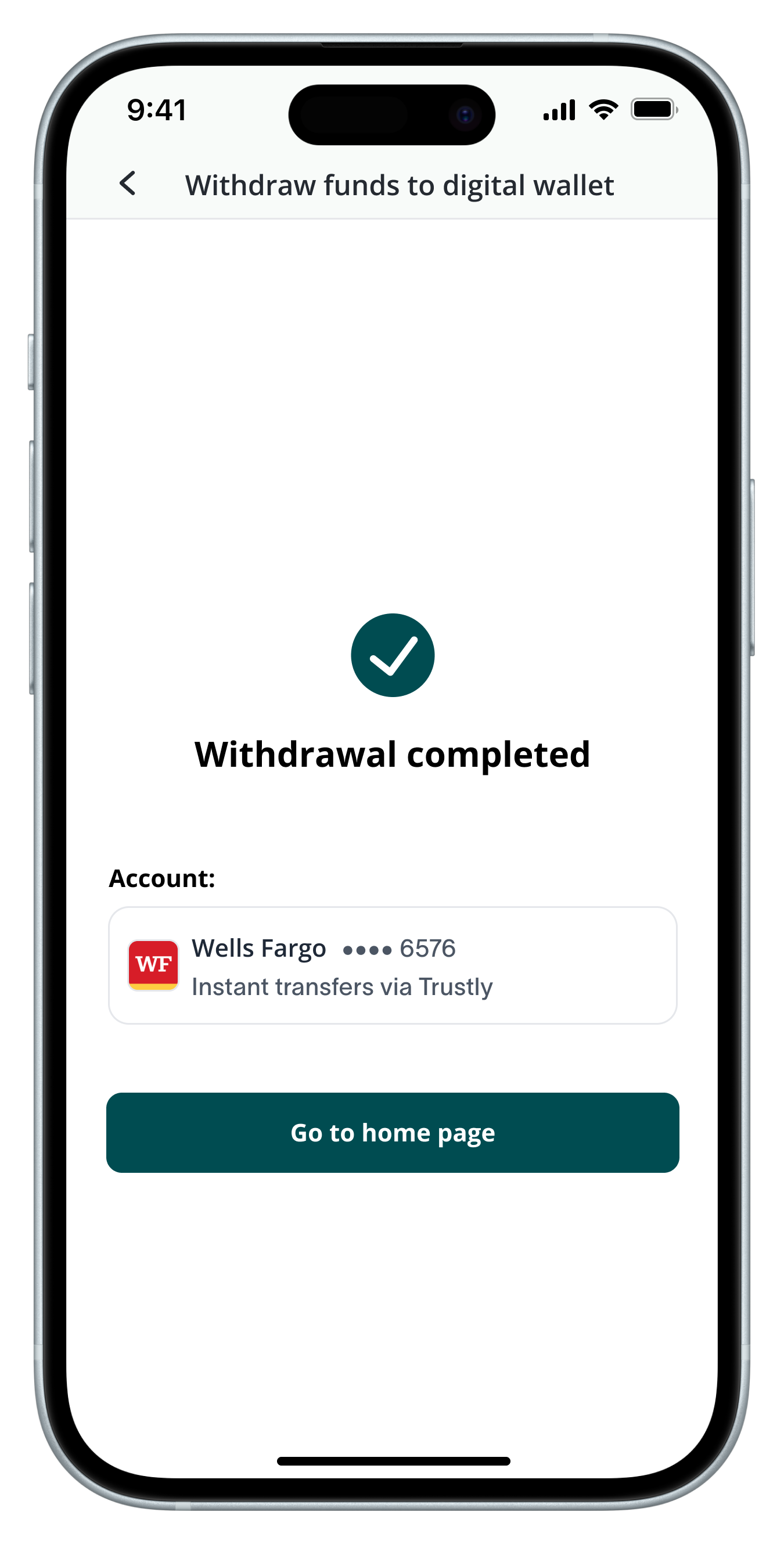
Example Get Transaction response (abbreviated)
{
"transaction": {
"transactionId": "1002580075",
"payment": {
"account": {
"name": "Adv Plus Banking",
"type": 1,
"accountNumber": "3254",
},
"paymentProvider": {
"paymentProviderId": "051000017",
"name": "Bank with real-time payments",
"instantPayoutAvailable": true
},
},
"statusMessage": "Authorized"
}
}
Creating Deferred Transactions with the Deposit API
With a valid transactionId
from an Authorization request, you can use the Trustly Deposit Transaction to initiate a Payout transaction to a user's bank account.
To initiate the request, pass in the following inputs:
transactionId
: Authorization Transaction ID retrieved from a Trustly Deferred Authorization.amount
: The amount to be sent. Required if the Authorization Transaction amount was0.00
. If not passed, the full amount of the Authorization Transaction will be used.merchantReference
: A specific merchant reference for this deposit. For example, this could be a merchant order number or the same merchant reference value used in the original establish call.instantPayoutRequest
: Whether an instant payout is requested for this transaction.
If successful, the response of this call will be an authorized Payout transaction. Updates to the status of the transaction will be sent via Event Notification.
Example Request
POST /transactions/1002613662/deposit
amount=25.00&merchantReference=f9c28cd0-bca1-47cb-a776-238b621e66cb&instantPayoutRequest=true
Example Response (Abbreviated)
{
"transaction": {
"transactionId": "1002613685",
"transactionType": 6,
"originalTransactionId": "1002613662",
"payment": {
"paymentId": "1002580220",
"paymentType": 2
},
"currency": "USD""CAD",
"amount": "25.00",
"paymentProviderTransaction": {
"paymentProviderTransactionId": "ptx-M17zSYl0YI7lzHC02tsFnF9g-sbx",
"status": 10,
"statusMessage": "Established",
"instantSettle": true, // OPTIONAL, based on merchant configuration
"paymentProcessor": {
"paymentProcessorId": "100000001"
}
},
"status": 2,
"statusMessage": "Authorized",
"createdAt": 1556922524381,
"processedAt": 1556922524393,
"completedAt": 1556922524621,
"updatedAt": 1556922524622,
"ppTrxId": "ptx-CquvTsAaPDvaSB6ywZ2krXGS-sbx",
"merchantReference": "f9c28cd0-bca1-47cb-a776-238b621e66cb",
"paymentProcessorType": "USRTP",
"statusCode": "AC118",
"recordVersion": 1
}
}
The
instantSettle
parameter being present in the response body is configurable by your Engagement Manager.
Updated 3 months ago