Payments
Integrating Trustly Pay into your application allows users to authenticate with their bank and authorize transactions seamlessly. This tutorial will guide you through the process of creating a bank authorization, retrieving bank and user data, initiating payment transactions, and handling event notifications. By the end, you'll be able to offer a secure and efficient payment experience for your users.
Overview
Conceptually, there are two major steps:
First, request a mandate from the consumer, allowing access to their bank account. This mandate is commonly referred to as an Authorization and is identified via a transactionId, and can thereafter be used for multiple payments.
Trustly offers 3 SDK's to support the front-end user interactions. These SDKs support JavaScript, iOS, and Android. The SDK has 2 main methods: selectBankWidget and establish, which support different user interfaces (so you only need to implement one).
Second, perform the specific payment or payments against that mandate. These may include funds capture preauthorization, funds capture, and/or funds deposit (payout).
Optional: Retrieve user and bank account information to represent it to the user as necessary.
Steps Breakdown
The steps to integration Trustly Pay can be further broken down into the following sections.
This step is initiated by calling the establish
method of the SDK and launches the Trustly Online Banking interface which allows a User to authenticate with their Bank and authorizes you to use that bank for payment transactions. The successful call results in the mandate aka authorization represented by the returned transactionId
. All subsequent API calls that result in the actual payment will be against this transactionId
.
With a valid transactionId
, you can use the Trustly Get Transaction API and Get Financial Institution User API to retrieve information from the User's selected bank to display, verify, or pre-fill fields on your site or application.
When the User requests to make a payment or requests a payment from you, you can use the Trustly Capture API or Deposit API, passing the previously obtained transactionId
, amount
, and a merchantReference
that will be passed back to you in any event notifications, allowing you to manage the context associated with the payment request. You can also optionally first perform a capture preauth request, which performs the risk analysis and funds availability for the indicated amount, so that you can perform the actual Capture later.
Implement Trustly Event Notifications to receive transaction status updates in real time and message your customers accordingly. Some relevant cases are when the payment transaction returns a PENDING or ERROR response. Also an authorization can become invalidated, for example, if the user cancels the mandate or it expires. If that happens, you'll want to send the User back to Online Banking to select a new Bank or refresh their Bank Authorization.
Integration Options and Branding Requirements
Trustly offers two types of user experiences for its Online Banking solution: Trustly Widget and Trustly Lightbox.
The Trustly Widget represents a bank-selection shortcut. It is shown in-line on your page and highlights the most popular bank while adding the capability to quickly search for a bank not expressly represented. Selecting one of the buttons on the Widget opens the Trustly Lightbox, where the User can sign in and authorize their account for use.
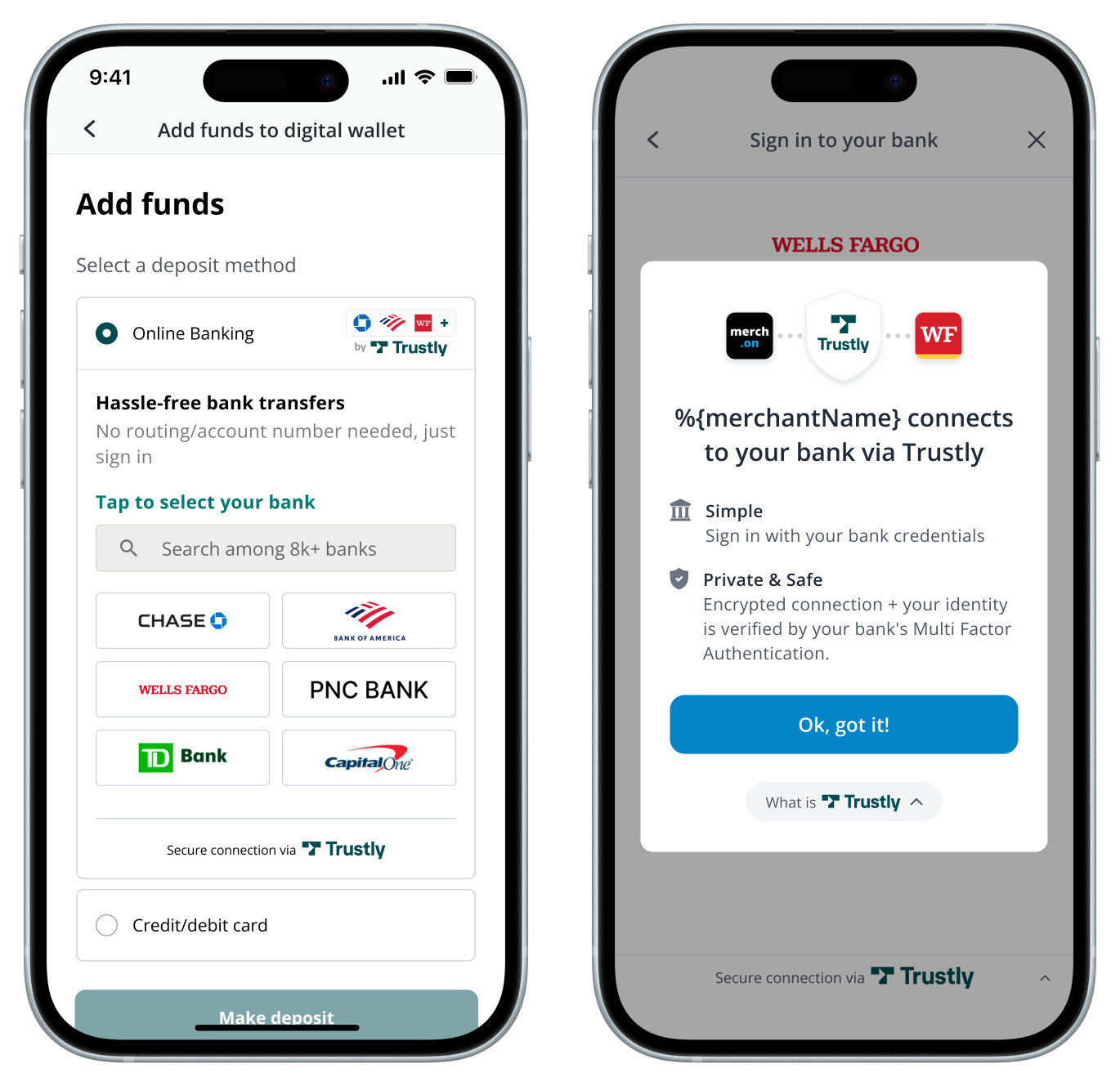
Alternatively, you can trigger the Trustly Lightbox using your own button. The Trustly Lightbox opens over your existing page in a mobile environment and presents a longer list of banks.
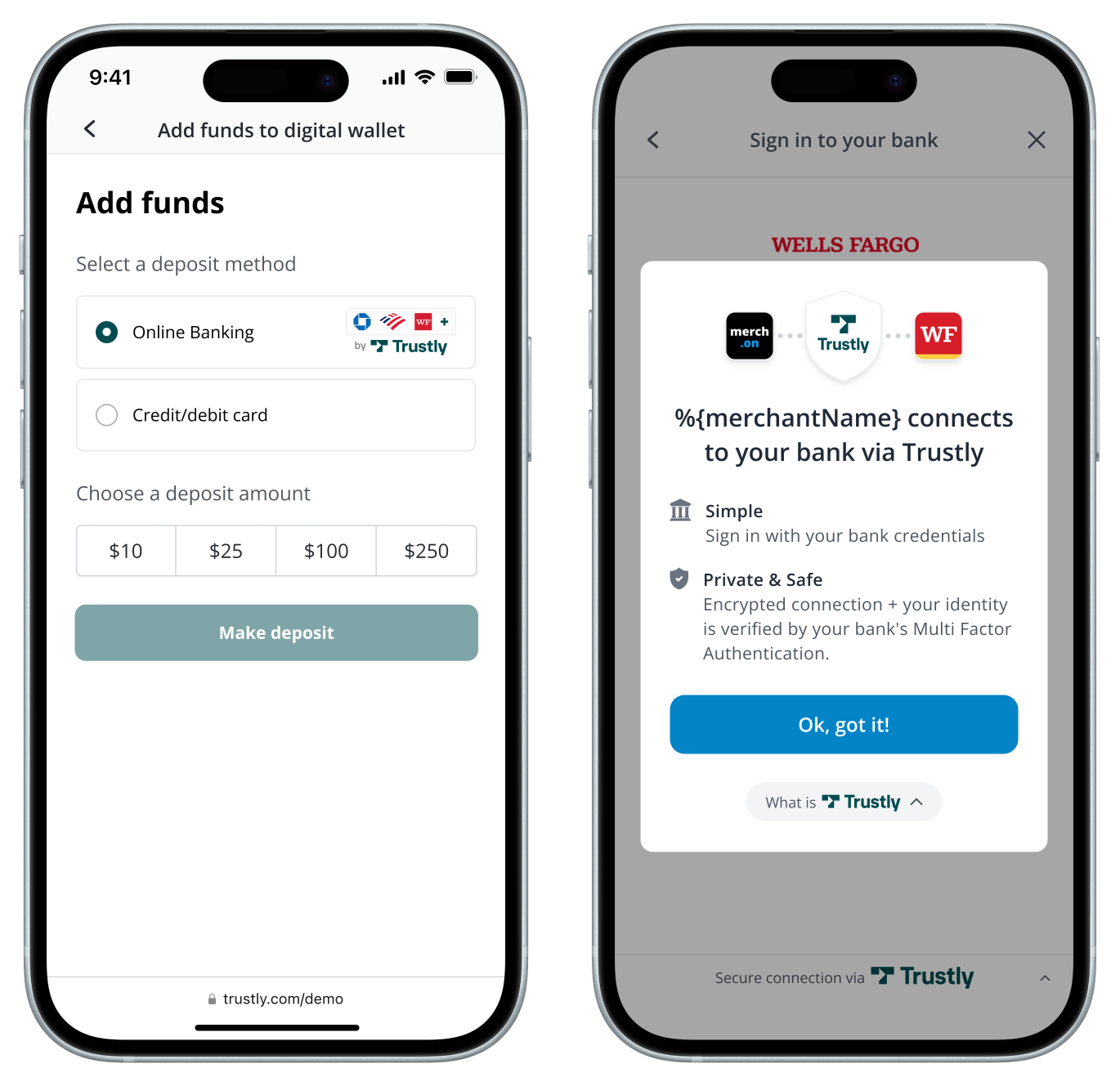
In addition to determining if the Select Bank Widget or opening the Trustly Lightbox directly is the best option for you, Trustly has a number of Branding Requirements to consider. If you have any questions or specific requirements, please work with your Trustly team or email [email protected].
Testing
Trustly offers a Demo Bank in the Sandbox environment that can be used to trigger a number of testing scenarios. You access the Demo Bank, search for "Demo Bank" in the 'Select your bank' screen of the Trustly Lightbox. To simulate errors when using the Demo Bank, use the phrases below in the password field to generate errors.
See the Testing Guide for more details
Steps
See the linked guides below to get started implementing your Trustly payments solution.
Updated about 1 year ago